Basic way to create a thread
- Implement Runnable
- Extends Thread
Life cycle
- New: A thread that has not yet started is in this state.
- Runnable: A thread executing in the Java virtual machine is in this state.
- Blocked: A thread that is blocked waiting for a monitor lock is in this state.
- Waiting: A thread that is waiting indefinitely for another thread to perform a particular action is in this state.
- Timed Waiting: A thread that is waiting for another thread to perform an action for up to a specified waiting time is in this state.
- Terminated: A thread that has exited is in this state.
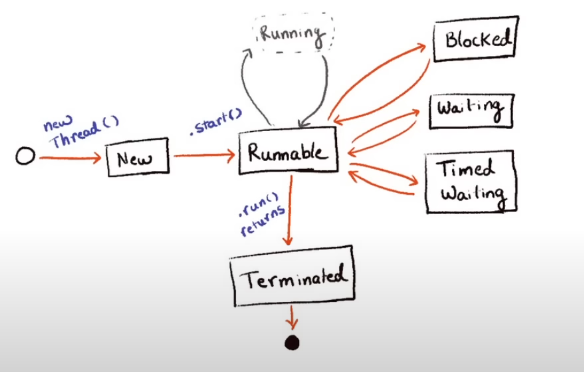
Joining Threads
Joining threads is a mechanism that allows one thread to wait for the completion of another thread before it continues its execution. When a thread invokes the join()
method on another thread, it will pause its execution until the other thread completes (terminates).
The join()
method is defined in the Thread class and has three variants:
join()
– Waits indefinitely for the thread to diejoin(long millis)
– Waits for the thread to die for the specified number of millisecondsjoin(long millis, int nanos)
– Waits for the thread to die for the specified number of milliseconds plus nanoseconds
Concurrency vs Parallelism
Parallelism is
About physically executing multiple tasks at the exact same moment (requires multiple processors)
- 2 threads are running in 2 cords
- Running many different tasks at the same time
- By definition, needs multiple cores
Concurrency is
About dealing with multiple tasks by switching between them (even on a single processor)
- 10 threads running on 1 core
- Multiple tasks in progress at the same time (but not necessarily executing them simultaneously)
Concurrency Problems
- Don’t have shared state
- Share only immytable values
- Use synchonization
-> No concurrency issue
Race Condition
occurs when multiple threads access and modify shared data simultaneously, leading to unpredictable or incorrect results
How to avoid
1. Synchronization
Make sure 2 threads don’t simultaneously access a critical data element
Only 1 thread can execute the block at the same time
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
2. Lock Mechanisms
Deadlocks
- Mutiple threads are waiting for other threads
- The dependency is circular
Example scenario:
- Thread A holds lock 1 and waits for lock 2
- Thread B holds lock 2 and waits for lock 1
- Neither thread can proceed
Liveness
Similar to deadlocks, but threads are not blocked—they’re actively running while making no progress because they keep responding to each other’s actions.
Starvation
Occurs when a thread is denied access to resources it needs for extended periods, preventing it from making progress.
Volatile Keyword
- Changes made by one thread to a volatile variable are immediately visible to other threads
- Marks a variable as do not cache
- Every read from a thread í directly from the main memory
- Every write from a thread is made directly to the main memory
- Only guarantees visibility, not atomicity
- Only one thread writes, others read
Amtomic Class
Thread Local
- Scope is per-thread
- Each thread just sees its own thread local variable
Leave a Reply